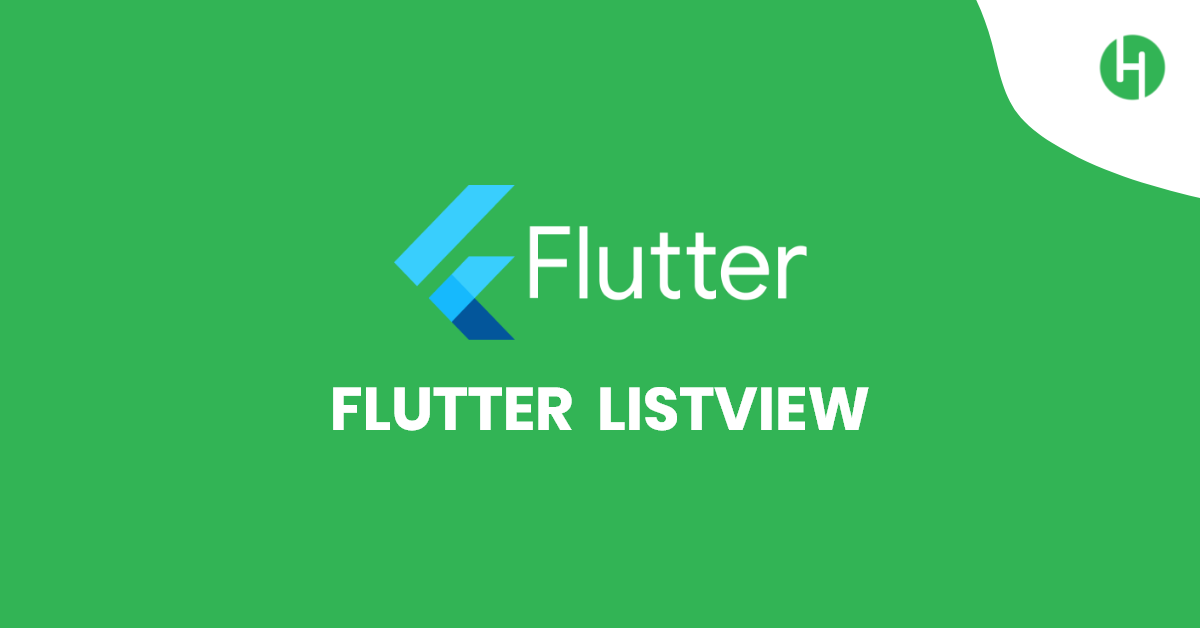
Flutter is an open source Google’s UI toolkit for building beautiful, natively compiled applications for mobile, web, desktop, and embedded devices from a single codebase. Flutter is fast in the development process, can give native performance and can have flexible and adoptable ui components. Here, in this blog we are going to look at ListView widget in detail.
“ListView Widget comes in when you are to show a list of scrolling items on your application.”
ListView is the most commonly used scrolling widget. It displays its children one after another in the scroll direction. In the cross axis, the children are required to fill the ListView. This ListView widget will be so helpful when you are to show different widgets in a scrollable list. The list can either be in vertical or horizontal direction. All you need to do is play with some words inside the ListView widget.
There are four options for constructing a ListView: (Here, we will cover only the first three methods.)
- The default constructor takes an explicit List<Widget> of children. This constructor is appropriate for list views with a small number of children because constructing the List requires doing work for every child that could possibly be displayed in the list view instead of just those children that are actually visible.
- The ListView.builder constructor takes an IndexedWidgetBuilder, which builds the children on demand. This constructor is appropriate for list views with a large (or infinite) number of children because the builder is called only for those children that are actually visible. If you have long lists of items or you are creating a list dynamically, you can use this version of ListView Widget.
- The ListView.separated constructor takes two IndexedWidgetBuilders: itemBuilder builds child items on demand, and separatorBuilder similarly builds separator children which appear in between the child items. This constructor is appropriate for list views with a fixed number of children. This version is just the same as ListView.builder but it adds white space between each list item with the Divider widget.
- The ListView.custom constructor takes a SliverChildDelegate, which provides the ability to customize additional aspects of the child model. For example, a SliverChildDelegate can control the algorithm used to estimate the size of children that are not actually visible.
Let’s dive into code.
1. ListView
– It takes a children attribute with a list of items for the ListView. And those items will be widgets. Here, I have three ListTile widgets.
ListView( children: [ ListTile( leading: Icon(Icons.arrow_right), title: Text("January"), ), ListTile( leading: Icon(Icons.arrow_right), title: Text("February"), ), ListTile( leading: Icon(Icons.arrow_right), title: Text("March"), ), ), ],
Output Looks like This:

2. ListView.builder
– It will have an itemCount attribute, which tells ListView how many items will be in the ListView. Additionally, it will have an itemBuilder function with BuildContext and iterator and returns a Widget. Here, the itemBuilder is returning a ListTile widget.
List months = ['January', 'February', 'March', 'April', 'May']; ListView.builder( itemCount: months.length, itemBuilder: (BuildContext context, index) { return ListTile( leading: Icon(Icons.arrow_right), title: Text(months[index]), ); }, ),
Output Looks like This:

3. ListView.separated
-It is very similar to the ListView.builder but it will insert some widget like Divider (in this example) as a separator between each of the items. The separating widget will be in the separatorBuilder function.
List months = ['January', 'February', 'March', 'April', 'May']; ListView.separated( itemCount: months.length, separatorBuilder: (BuildContext context, int index) => const Divider( color: Colors.blue, ), itemBuilder: (BuildContext context, index) { return ListTile( leading: Icon(Icons.arrow_right), title: Text(months[index]), ); }, ),
Output Looks like This:

Let’s have more control over the ListView widget.
- You can make your List scroll vertical or horizontal with the following command.
scrollDirection: Axis.horizontal, scrollDirection: Axit.vertical,
- If you want to just reverse your list.
reverse: true,
- If you want to adjust physics on your list.
physics: NeverScrollableScrollPhysics(),
- If you want to keep the list items alive when they are off screen.
addAutomaticKeepAlives: false,
You can have in detail information on the ListView widget in this official Flutter Widget Of The Week from Google Flutter Team.